Guide: Setup Chatbot in Next.js
A step-by-step guide to setup a chatbot in Next.js using Langbase components.
Prerequisites: Create an AI memory & AI agent
This guide assumes you have already created an AI memory and agent on Langbase. If you haven't, please follow these guides:
In this guide, we will learn how to setup a chatbot in Next.js using Langbase components. We will:
- Langbase SDK and components: Install Langbase SDK and components to your Next.js app.
- API route: Create an API route to call our AI agent.
- Chatbot component: Setup chatbot component in your Next.js app.
Let's get started.
Step #1Install Langbase SDK and components
We will use Langbase SDK to call our AI agent and Langbase components to import a pre-built chatbot component.
npm i langbase @langbase/components
Step #2Create an API route
Go ahead and create an API route in your Next.js app where we will request our AI agent pipe using Langbase SDK. Add the following code in the API route:
import { NextRequest } from 'next/server';
import { Langbase } from 'langbase';
const langbase = new Langbase({
apiKey: process.env.LANGBASE_API_KEY! // Your Langbase API key
});
export async function POST(req: NextRequest) {
const options = await req.json();
const { stream, threadId } = await langbase.pipe.run({
...options,
name: 'REPLACE-WITH-PIPE-NAME' // e.g. 'ask-xyz-docs'
});
return new Response(stream, {
status: 200,
headers: {
'lb-thread-id': threadId ?? ''
}
});
}
Please replace REPLACE-WITH-PIPE-NAME
with the name of your AI agent pipe.
Step #3Setup chatbot component
First we need to import the CSS for the chatbot component. Add the following code in your root layout:
import '@langbase/components/styles'
Now go ahead and import the chatbot component in the root page of your Next.js app:
'use client';
import { Chatbot } from '@langbase/components';
<Chatbot
apiRoute="<REPLACE-WITH-API-ROUTE>" // e.g. '/api/chat'
suggestions={[
{
title: `Explain how to get started in easy steps`,
prompt: `Explain how to get started in easy steps?`
},
{
title: `How do I create an API key?`,
prompt: `How do I create an API key?`
},
{
title: `What are the supported providers?`,
prompt: `What are the supported providers?`
},
{
title: `How do I reset my password?`,
prompt: `How do I reset my password?`
}
]}
/>
You can read complete API reference of Chatbot
component here.
Step #4Test your chatbot
Go ahead and run the Next.js app. You should see the chatbot on the bottom right corner of your app.
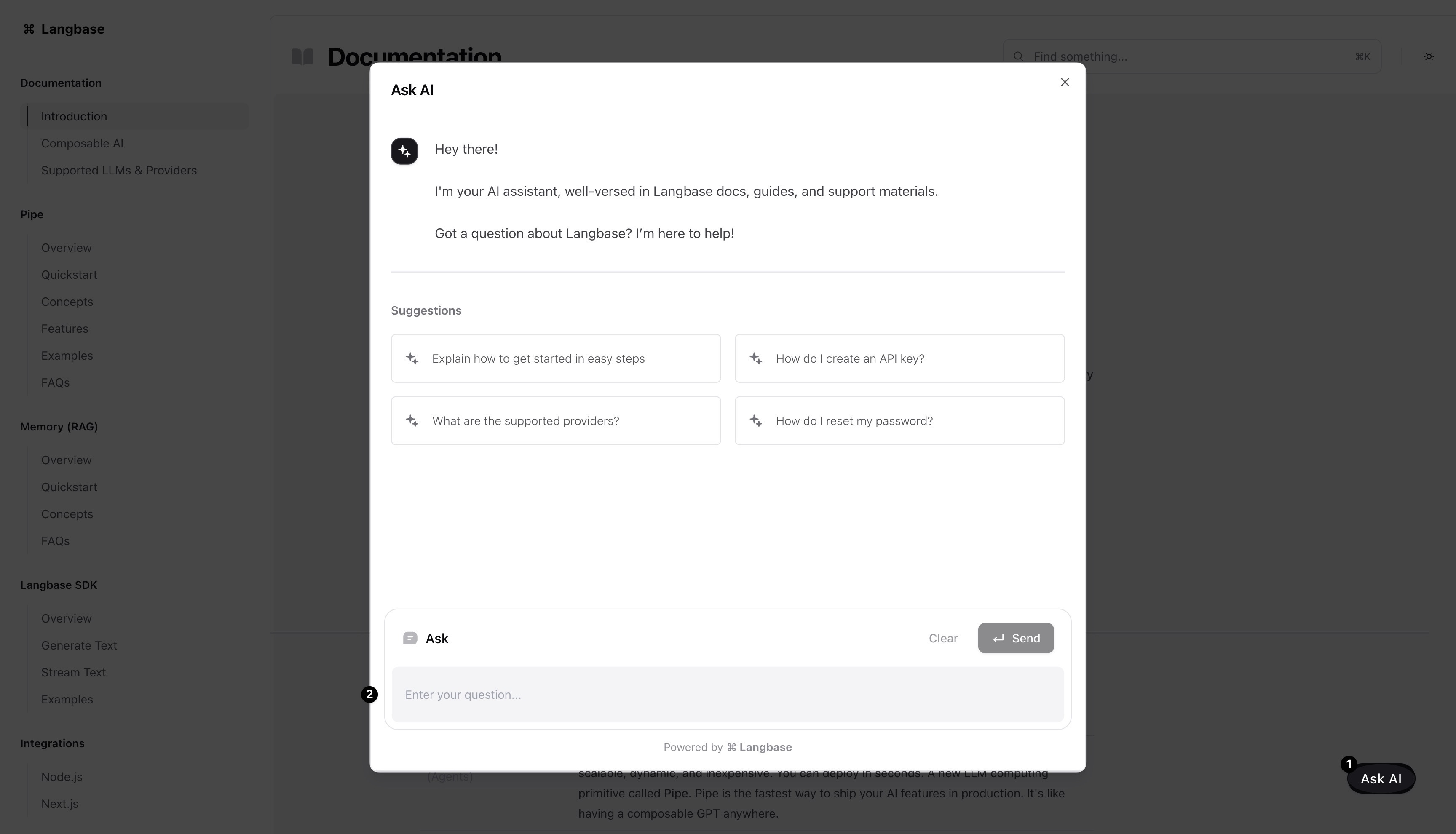
You can now ask questions from your documentation and the chatbot will answer them using the Docs agent.
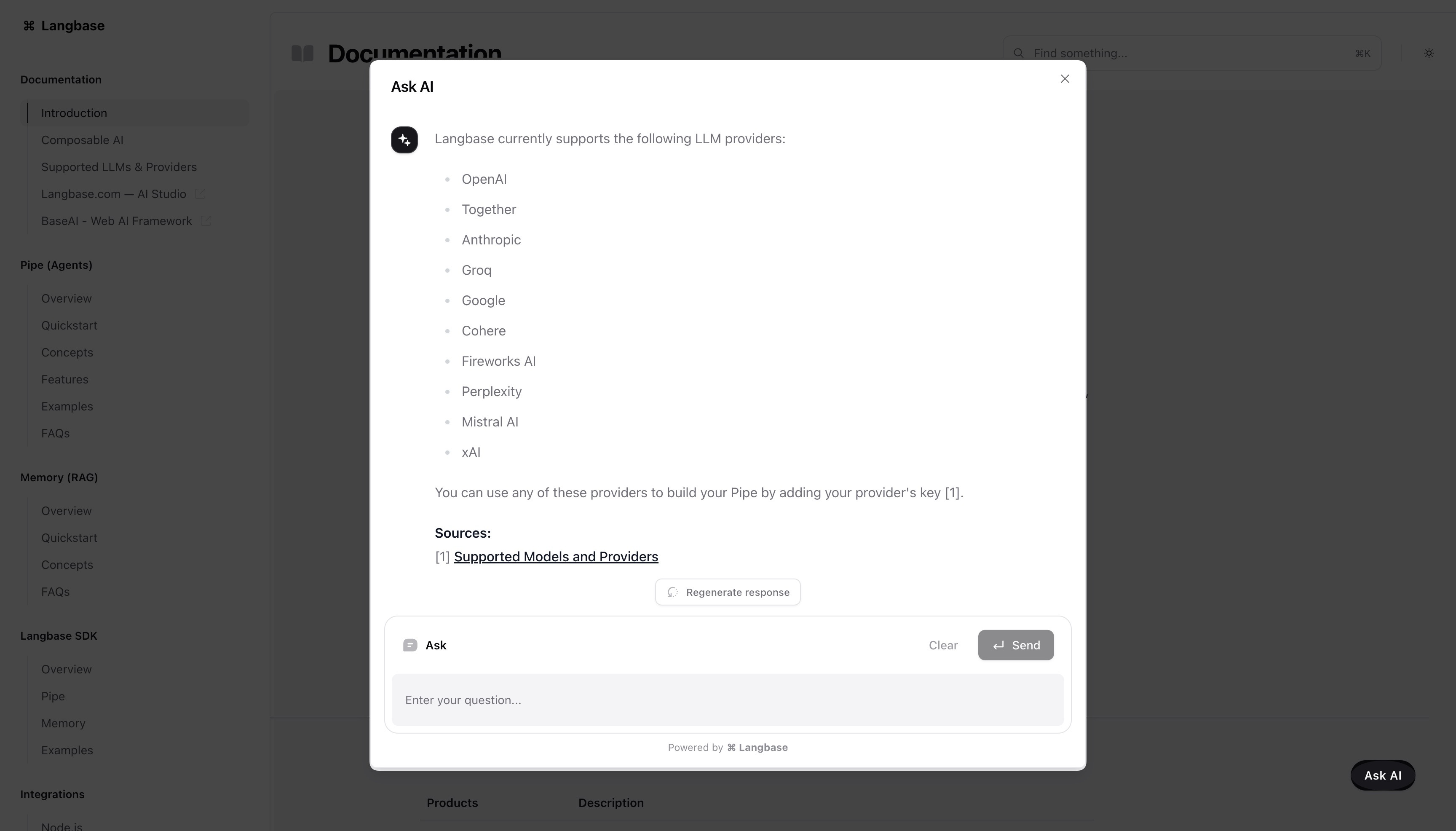
Wrap up
That's all you need to add AI in your docs using Langbase. To summarize, we:
The chatbot is now ready to answer questions from your documentation.
Next Steps
- Build something cool with Langbase.
- Join our Discord community for feedback, requests, and support.